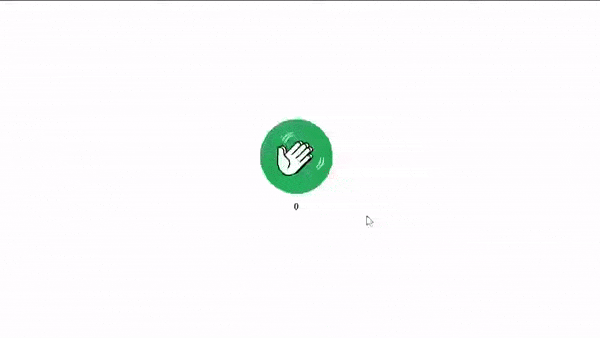
WordPress is a master of its own class and the number of people using it keeps on increasing day by day. In this post, I will help you build a Simple Clap Button Plugin that you can integrate into your posts, just like the one you see on the left side of this post. According to the hostingtribunal.com :
WordPress powers 35% of the internet in 2020, an increase of 2% compared to early 2019 and a 4% rise from the previous year.
If you are among that 35%, then it is possible that you own a blog or even a website. You would be posting a lot of interesting articles but how to identify whether a visitor likes them or not?
Well! A Clap Button π perhaps.
Appreciation matters, because it gives an extra layer of confidence to the writers. So, without any further ado, let’s get started.
Things to know beforehand
- Knowledge on HTML, CSS, and JS (all basics)
- Know how PHP works for server-side scripting
- Aware of WordPress functions and AJAX calls
It’s fine even if you are new to WordPress or any of those mentioned above. Just make sure you follow all the steps to get the final result you desire.
AJAX and WordPress
WordPress, by default, uses AJAX but only in the admin screen. An example of an AJAX call would be the auto–save functionality in the WordPress editor since it does not require a page refresh while saving because of Asynchronous calls.
AJAX is commonly used with jQuery and since WordPress comes with the jQuery library loaded, we don’t need to include it explicitly.
Also, when a user triggers an AJAX request, it is first passed to the admin-ajax.php
file in the wp-admin
folder. This file is available in WordPress by default.
Creating a WordPress AJAX plugin
Before we start creating the plugin, let’s look at the features that we will be building:
- Both logged-in and logged-out users can clap for the post.
- It keeps the count of claps for individual posts.
- The Clap counter is automatically updated in the frontend.
- You can customize the Clap button based on your needs once you have built it successfully.
If you only want the logged in users to clap for your posts, then I’ll be helping you with that as well in the upcoming steps.
Step 1: Create An Empty Plugin
Navigate to the folder wp-content/plugins/
in your WordPress installation and create a new folder called claps-for-posts
. Make sure to give a unique name so that it should not contradict another plugin’s name that you already use.
You can navigate to the wp-content/plugins
folder in two ways.
- Using an FTP client that is connected to your WordPress (Refer here)
- Using the File Manager provided by your Hosting Provider.
I would recommend you to use an FTP client since its the safer option. After creating a folder, create a file named claps-for-posts.php and add the following lines.
<?php
/**
* Plugin Name: Applause for Posts
* Plugin URI: https://www.subbuswaroop.com
* Description: This plugin adds a applause feature for every post
* Version: 1.0.0
* Author: Subbu Swaroop
* Author URI: https://www.subbuswaroop.com
* License: GPL2
*/
Note: Make sure that the file name and the folder name are the same.
These are just comments that don’t have any impact on the WordPress code. They are just for reference purposes only. Make sure to modify the details that represent your plugin and yourself.
Once you save the file, refresh the Plugins page in the WordPress admin. You should see a plugin with the “Plugin Name” you have given. If not, log out of WordPress and log in again. But do not forget to activate the plugin before going to Step 2.
Step 2.1: Make Post Template Ready
Now that you have successfully created an empty plugin (KUDOS!!! for that ), let’s create the template part of our code. In our use case, we will be focusing on adding a Clap button for every post in a blog.
<div class="clapsdiv">
<div class="clapforpost">
<div id="postclapped">
<p>+1</p>
</div>
<div id="tophand" onclick="clapforme(this)">
<?php
echo '<a class="user_clap" data-nonce="' . $nonce . '" data-post_id="' . $post->ID . '" href="' . $link . '">'; ?>
<!-- SVG code should be present here
Please go to "https://codepen.io/subbuswaroop/pen/rNOVNga" to get the SVG code corresponding to this <div id="tophand">.
-->
<?php echo '</a>';
?>
</div>
<div id="bottomhand">
<!-- SVG code should be present here
Please go to "https://codepen.io/subbuswaroop/pen/rNOVNga" to get the SVG code corresponding to this <div id="bottomhand">.
-->
</div>
</div>
<div class="showclaps">
<p>
<?php
$claps = get_post_meta($post->ID, "claps", true);
$claps= ($claps== "") ? 0 : $claps;
?>
<span id='clap_counter'><?php echo $claps?></span><br>
</p>
</div>
</div>
<script>
function clapforme(element) {
var bottomhand = document.getElementById("bottomhand");
var likepost = document.getElementsByClassName("user_clap");
var claps = document.getElementById("postclapped");
var vibrations1 = document.getElementsByClassName("cls-vibration1");
var vibrations2 = document.getElementsByClassName("cls-vibration2");
for (let i = 0; i < vibrations1.length; i++) {
vibrations1[i].classList.add("vibration-top");
vibrations2[i].classList.add("vibration-bottom");
}
element.classList.add("applauseanimation", "disableclaps");
bottomhand.style.backgroundColor = "grey";
claps.classList.add("addclaps");
}
</script>
I will be adding the above code to content-single.php
in my theme. You can place them in single.php
or any other template file where you need the Clap button to be present. Since I’ll be using SVG’s, I have provided a link to my CodePen, where you can get the SVG code for the corresponding div
.
Step 2.2: Adding the CSS
Since we are building a Clap button that should look like the one mentioned earlier, CSS is mandatory. You can refer to my CodePen, copy the CSS code and place it in your main CSS file.
Note: Make sure that your code does not have any selectors that contradict the name I have used in my code.
Step 3: Handling The AJAX Call Without JS
Now, open the plugin file we have created and append the following code.
add_action("wp_ajax_user_clap", "user_clap");
add_action("wp_ajax_nopriv_user_clap", "user_clap");
// define the function to be fired for logged in users
function user_clap()
{
// nonce check for an extra layer of security, the function will exit if it fails
if (!wp_verify_nonce($_REQUEST['nonce'], "user_clap_nonce")) {
exit("Nonce sense");
}
// fetch clap_count for a post, set it to 0 if it's empty, increment by 1 when a click is made
$clap_count = get_post_meta($_REQUEST["post_id"], "claps", true);
$clap_count = ($clap_count == '') ? 0 : $clap_count ;
$new_clap= $clap_count + 1;
// Update the value of 'clap' meta key for the specified post, creates new meta data for the post if none exists
$clap = update_post_meta($_REQUEST["post_id"], "claps", $new_clap);
// If above action fails, result type is set to 'error' and clap_count set to old value, if success, updated to new_like_count
if ($clap === false) {
$result['type'] = "error";
$result['clap_count'] = $clap_count ;
} else {
$result['type'] = "success";
$result['clap_count'] = $new_clap;
}
// Check if action was fired via Ajax call. If yes, JS code will be triggered, else the user is redirected to the post page
if (!empty($_SERVER['HTTP_X_REQUESTED_WITH']) && strtolower($_SERVER['HTTP_X_REQUESTED_WITH']) == 'xmlhttprequest') {
$result = json_encode($result);
echo $result;
} else {
header("Location: " . $_SERVER["HTTP_REFERER"]);
}
// don't forget to end your scripts with a die() function - very important
die();
}
// define the function to be fired for logged out users
function please_login()
{
echo "You must log in to like";
die();
}
If you are confused after seeing the above code, then its perfectly fine. The only thing you need to look for is the first two lines of the code.
add_action("wp_ajax_my_user_like", "user_clap");
add_action("wp_ajax_nopriv_my_user_like", "user_clap");
The first one works for users who are logged in to WordPress and the second one works for those who are logged out. I have called the same function “user_clap” for both since I prefer everyone to clap for mine. You can change it to the following if you want only logged in users to perform a clap.
add_action("wp_ajax_my_user_like", "user_clap");
add_action("wp_ajax_nopriv_my_user_like", "please_login");
For now, the “please_login” function does not do much. You can alter it based on your own logic. For browsers that have Javascript disabled, clicking on the Clap button will refresh the page but the clap count will be incremented by 1.
Note: A user can only clap once.
Step 4: Adding Javascript Support
For AJAX to work with WordPress, you need to enqueue jQuery library and your custom JS file. Append the following to the main plugin file.
add_action('init', 'script_enqueuer');
function script_enqueuer()
{
// Register the JS file with a unique handle, file location, and an array of dependencies
wp_register_script("clap_script", plugin_dir_url(__FILE__) . 'clap_script.js', array('jquery'));
// localize the script to your domain name, so that you can reference the url to admin-ajax.php file easily
wp_localize_script('clap_script', 'myAjax', array('ajaxurl' => admin_url('admin-ajax.php')));
// enqueue jQuery library and the script you registered above
wp_enqueue_script('jquery');
wp_enqueue_script('clap_script');
}
Now let us create a custom Javascript file “clap_script.js” inside the root of the plugin folder where your main plugin file is and then add the following code to it.
jQuery(document).ready(function () {
jQuery(".user_clap").click(function (e) {
e.preventDefault();
post_id = jQuery(this).attr("data-post_id");
nonce = jQuery(this).attr("data-nonce");
jQuery.ajax({
type: "post",
dataType: "json",
url: myAjax.ajaxurl,
data: { action: "user_clap", post_id: post_id, nonce: nonce },
success: function (response) {
if (response.type == "success") {
jQuery("#clap_counter").html(response.clap_count);
jQuery(".user_clap").removeAttr("href");
} else {
alert("Your like could not be added");
}
},
});
});
});
This AJAX code will be used to update the count of the claps without refreshing the page. If you have followed every step from the beginning, then you should be getting a fully functional Clap button floating on the left side of your post.
Conclusion
I hope you are happy with the Clap button. If so, you can clap for this post as well to show your support :D.
If you have any doubts or got stuck somewhere, post it in the comments and I’ll gladly answer them.
Signing off for now.
Arigato Gozaimasu π
Wow,interesting post Subbu Swaroop.
Worth experimentingπ
really lovely plugin that I will love to see among other plugins on wordpress
Thanks Ajetomobi π
Can we get the plugin install files for those who just want it installed? π thanks
Will keep that in mind Vish. Until then, u can use the code and customise it for yourself π
Hey Subbu great work buddy,
can you create the video tutorial for this it will really help me or direct plugin to install
Thanks, Sachin. I would love to do a video tutorial but I’m not a YouTuber π
I might make it as a plugin anytime sooner. Until then, you can follow the steps that I have mentioned and create it yourself. It will be a piece of cake.
HI thanks for ur plugin , i have one issue after clicking the count is getting incremented but when we go back and open same post again it is showing 0 again.please help with this.
Thank you
Hi Ramesh,
The following line should update the post_meta column.
$clap = update_post_meta($_REQUEST[“post_id”], “claps”, $new_clap);
You can check the table if its getting updated whenever you press the clap button. Let me know if you face any more issues.
Cheers π
Hi swaroop,
Thanks for your reply.
I did’nt get your point can you please give it in detail the clap count is not getting updated it is showing count 0.
Thank you.
Hi Ramesh,
Whenever someone clicks on the clap button, I am storing the count in the table “post_meta” under the column name “claps”.
You can check in your phpmyadmin database to see if the table has the column “claps”.
Also, can you go through the code once again to check if you have missed any important step?
Cheers π
I have used your codes and followed proper steps your code works fine but neither storing any data into database nor increasing the values, when i refreshed the page again showing 0.
Hey Lokesh, are you getting any errors in console by any chance?